검색결과 리스트
IT(Old)에 해당되는 글 41건
- 2008.01.22 플래시 사용예제
- 2008.01.22 쿠키사용예제
- 2008.01.22 세션과(session) 플래시(flash) 기능 팁
- 2008.01.21 모델 데이타의 검증
- 2008.01.21 rails console사용하기 2 (activeRecord의 수정,삭제사용법)
- 2008.01.21 rails console사용하기 1 (activeRecord의 find 사용법)
- 2008.01.21 Model 명명규칙 관례를 따르지 않을 경우
- 2008.01.21 rails 플러그인 목록 주소(http://agilewebdevelopment.com/plugins/list)
- 2008.01.21 각 mode별로 start 방법
- 2008.01.21 migration 문법
글
플래시 사용예제
class UsersController < ApplicationController
def list
@users = User.find(:all)
@message = flash[:message]
end
def show
@user = User.find(params[:id])
end
def new
@user = User.new
end
def create
@user = User.new(params[:user])
if @user.save
flash[:message] = "새로운 사용자가 추가되었습니다."
redirect_to :action => 'list'
else
render :action => 'new'
end
end
end
설정
트랙백
댓글
글
쿠키사용예제
옵션들
:expire = 웹 브라우저가 쿠키를 얼마나 오랫동안 보관할 것인지를 지정하는 옵션이다. :expire 옵션에는 쿠키가 유효한 최종 날짜를 지정하면 된다.
(expire옵션이 생략되는 경우, 해당 쿠키는 웹브라우저가 종료되는 순간까지만 보관된다.)
:domain = 쿠키가 사용될 도메인을 지정하는 데 사용된다. 이 옵션이 생략되면 현재 사용되고 있는 웹 애플리케이션의 도메인이 사용되는데, 이런 경우 쿠키가 다른 서브 도메인과 공유되지 않는다. 예를 들어 현재의 웹 애플리케이션이 www.mysite.co.kr도메인을 사용하고 있다면, 이 서버에서 웹 브라우저에 저장한 쿠키는 help.mysite.co.kr 도메인을 사용하는 웹 애플리케이션으로는 보내지지 않는다.
(사용법 : :domain => ".mysite.co.kr")
:path = 쿠키가 사용될 URL의 디렉토리 경로를 지정하는 데 사용된다. 예를 들어 :path => "/search" 라는 옵션을 지정하면, 이 쿠키는 URL이 www.mysite.co.kr/search로 시작되는 경우에만 서버로 보내진다. 이 옵션이 생략되면, 쿠키의 :path값으로 현재 페이지의 디렉토리 경로가 사용된다.
:secure = :secure => true 옵션을 사용하면, 쿠키가 https프로토콜이 사용되는 경우에만 보내지게 된다. HTTPS는 HTTP에 보안 기능이 추가된 프로토콜로, HTTPS를 사용하여 전송되는 모든 정보는 암호화되어 안전하게 전송된다.
class LoginController < ApplicationController
def login_form
reset_session
@login = cookies[:login]
end
def login
@user = User.find_by_login(params[:login])
cookies[:login] = {:value => @user.login, :expire => 30.day.from_now}
if @user && (@user.password == params[:password])
session[:user_id] = @user.id
redirect_to :action => "index"
else
flash[:error] = "로그인 ID나 비밀번호가 틀렸습니다!"
redirect_to(:action => "index")
end
end
def logout
reset_session
redirect_to(:action => "login_form")
end
def index
if session[:user_id]
render_text "#{User.find(session[:user_id]).name}님 환영합니다.!"
else
redirect_to :action => "login_form"
end
end
end
설정
트랙백
댓글
글
세션과(session) 플래시(flash) 기능 팁
1. session : session 메소드는 해시 객체를 리턴하는데, 이 해시가 바로 세션 데이터가 저장되는 장소이다.
사용자가 로그인 된 이후에는, sessoin[:user_id]이 현재 로그인된 사용자 ID를 리턴하게 된다. 만약 현재의 사용자가 로그인되어 있지 않다면, session[:user_id]는 nil을 리턴한다.
세션기능은 기본적으로 on 상태이기 때문에 이 기능을 필요치 않은 페이지에서는
각 컨트롤러 선언부 바로 밑에
session :off
을 사용하여 중지시킬수도 있다.
2. Flash : 플래시 기능이 유용한 것은 앞에서도 설명한 바와 같이 HTTP 프로토콜이 상태를 보존할 수 없는 프로토콜이기 때문이다. 플래시는 세션과는 다르게 웹 브라우저의 바로 다음 요청까지만 데이터를 보존한다. 즉 login 액션에서 플래시에 저장된 에러 메세지는 바로 다음 요청인 login_form 액션까지만 유지되고, 그 이후에는 자동으로 삭제된다. 플래시에 대한 자세한 내용은 5.5절 '플래시'에서 다루고 있다.
사용예제
class LoginController < ApplicationController
def login_form
reset_session
end
def login
@user = User.find_by_login(params[:login])
if @user && (@user.password == params[:password])
session[:user_id] = @user.id
redirect_to :action => "index"
else
flash[:error] = "로그인 ID나 비밀번호가 틀렸습니다!"
redirect_to(:action => "index")
end
end
def logout
reset_session
redirect_to(:action => "login_form")
end
def index
if session[:user_id]
render_text "#{User.find(session[:user_id]).name}님 환영합니다.!"
else
redirect_to :action => "login_form"
end
end
end
설정
트랙백
댓글
글
모델 데이타의 검증
=> 필드가 HTML폼의 체크박스가 선택되어 있음을 검증
2. validates_associated(object)
3. validates_confirmation_of(attribute, :message => "message")
=> HTML 폼에서 사용자의 실수를 방지하기 위해서 필드값을 중복하여 입력받을 때,
두 필드의 값이 일치함을 검증
4. validates_each attribute do |record, attr|
record.errors.add attr, "error_message" if condition
end
5. validates_exclusion_of(attribute, :in => enumerable_object, :message => "message")
=> 필드값이 지정된 배열 또는 구간의 원소중 하나가 아닐 것을 검증
6. validates_format_of(attribute, :with => /regular_expression/, :message => "message")
=> 필드값의 문자열이 지정된 정규식 패턴과 일치하는지를 검증
7. validates_inclusion_of(attribute, :in => enumerable_object, :message => "message")
=> 필드값이 지정된 배열 또는 구간의 원소중 하나임을 검증
8. validates_length_of(attribute, :maximum => max, :message => "message")
=> 필드값의 문자열 길이가 지정된 범위 내에 있음을 검증
9. validates_numericality_of(value, :message => "message")
=> 필드값이 숫자임을 검증
10. validates_presence_of(attributes, :message => "message")
=> 필드에 값이 지정되지 않았거나, 필드값이 빈 문자열인 경우 에러
11. validates_size_of(attribute, :maximum => max, :message => "message")
12. validates_uniqueness_of(attributes, :message => "message")
=> 필드값이 테이블의 다른 레코드와 중복되지 않음을 검증
설정
트랙백
댓글
글
rails console사용하기 2 (activeRecord의 수정,삭제사용법)
간단하다
@user = User.find(1)
@user.password = "aaa"
@user.save
2. 삭제
한개삭제
@user = User.find(3)
@user.destroy
여러개 삭제
@user = User.find(:all, conditions > ["id" >1])
@user.destroy_all
아이디로 삭제
User.delete(3)
User.delete([1, 2, 5])
조건별로 여러개 삭제
User.delete_all(["registered_on = ?", Date.today])
설정
트랙백
댓글
글
rails console사용하기 1 (activeRecord의 find 사용법)
ruby script/console
Loading development environment (Rails 2.0.2)
>>
위에서 >> 프롬프트는 루비의 irb프롬프트이다. 여기에서는 단순히 irb유틸리티를 실행한 것이 아니라
레일스 애플리케이션의 콘솔을 시작한 것이기 때문에, 앞에서 정의했던 User모델 클래스를 사용하는 것이
가능하다.
2. Data 입력
>> @user = User.new
=> #<User id: nil, name: nil, login: nil, password: nil, registered_on: nil>
>> @user.name = "jolaking"
=> "jolaking"
>> @user.login = "jola"
=> "jola"
>> @user.password = "jola"
=> "jola"
>> @user.registered_on = Date.today
=> Mon, 21 Jan 2008
>> @user.save
=> true ==> 저장이 성공되었음을 표시한다.
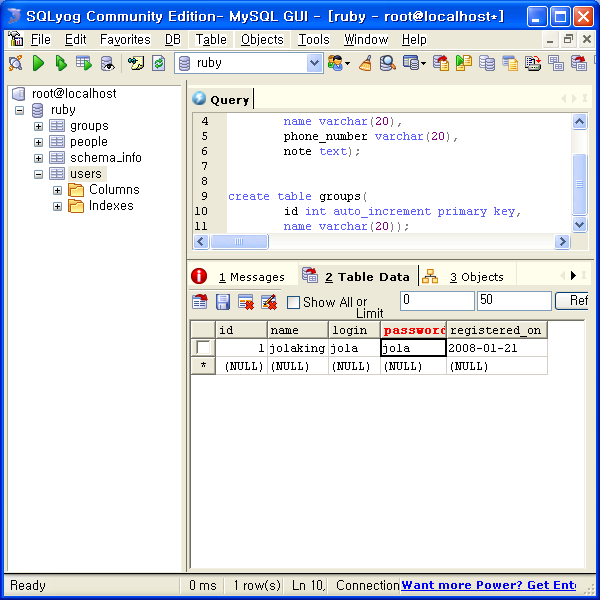
Data 확인
다른방법
>> @user = User.new(:name => "jolaking2",
?> :login => "jola2",
?> :password => "jola2",
?> :registered_on => Date.today)
=> #<User id: nil, name: "jolaking2", login: "jola2", password: "jola2", registe
red_on: "2008-01-21">
>> @user.save
=> true
>>
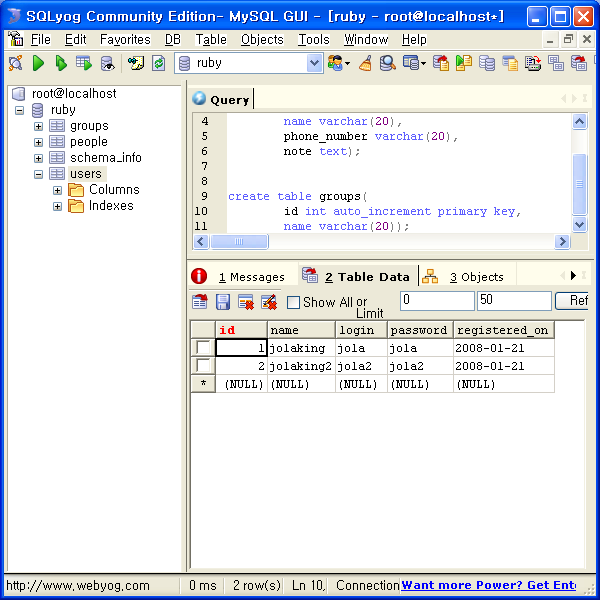
3. Data Retrieve
?> @user = User.find(1)
=> #<User id: 1, name: "jolaking", login: "jola", password: "jola", registered_o
n: "2008-01-21">
>> @user = User.find(2)
=> #<User id: 2, name: "jolaking2", login: "jola2", password: "jola2", registere
d_on: "2008-01-21">
>> @user = User.find(3)
ActiveRecord::RecordNotFound: Couldn't find User with ID=3
from c:/ruby/lib/ruby/gems/1.8/gems/activerecord-2.0.2/lib/active_record
/base.rb:1267:in `find_one'
from c:/ruby/lib/ruby/gems/1.8/gems/activerecord-2.0.2/lib/active_record
/base.rb:1250:in `find_from_ids'
from c:/ruby/lib/ruby/gems/1.8/gems/activerecord-2.0.2/lib/active_record
/base.rb:504:in `find'
from (irb):27
>>
주의할점 registered_on 메소드는 Date객체를 리턴하기 때문에 여기에서는 리턴된 Date 객체의
to_s 메소드를 호출하여 이를 문자열로 반환해야 된다.
find_by_xxxx 사용하기
?> @user = User.find_by_login("jola")
=> #<User id: 1, name: "jolaking", login: "jola", password: "jola", registered_o
n: "2008-01-21">
>> @user = User.find_by_login("jola2")
=> #<User id: 2, name: "jolaking2", login: "jola2", password: "jola2", registere
d_on: "2008-01-21">
>> @user = User.find_by_login("jola3")
=> nil >> 값이 없으면 nil
보다 복잡한 검색조건으로 찾기
?> str = "jola2"
=> "jola2"
>> @user = User.find(:first, :conditions => ["login=?", str])
=> #<User id: 2, name: "jolaking2", login: "jola2", password: "jola2", registere
d_on: "2008-01-21">
>>
여기서 :first는 첫번째 record의 return을 의미하고,
:all로 사용하면 배열로 여러 record를 받는다.
2가지 조건 이상 사용하면 다음과 같이 사용한다.
?> @user = User.find(:first, :conditions => ["login=? AND password=?" , "jola","jola"])
=> #<User id: 1, name: "jolaking", login: "jola", password: "jola", registered_on: "2008-01-21">
이쯤에서 사용되는 옵션을 정리하면
:first or :all = 첫번째 또는 전체
:conditions = Where 조건절 기술
:order = 정렬방식
:limit = 불러올 Record갯수
:offset = 지정된 숫자만큼 레코드를 건너뛰고, 그다음부터 리턴
:readonly = 리턴되는 레코드를 읽기 전용으로 만듦
conditions에 like 문을 사용하고자 한다면
:conditions = > ["login like ?", query + "%"]
직접 SQL문 입력가능
@group = Group.find_by_sql("SELECT ..... FROM aaa WHERE....");
다음에서 group값을 사용하고자 하면
@group[0].name 이런식으로
사용해야 된다.
설정
트랙백
댓글
글
Model 명명규칙 관례를 따르지 않을 경우
User < ActiveREcord:Base
set_table_name "user_tbl"
end
와 같이 강제로 table을 지정해 주어야 된다.
TIP : User 모델 클래스는 레일스 애플리케이션이 처음 구동될 때, 데이터베이스에 연결하여 users테이블의
필드 목록을 읽어들이게 된다. users 테이블의 필드 목록을 확인한 User 모델 클래스는 각 필드에 해당하는 접근자 메소드를 동적으로 정의하여 추가한다. 데이터 베이스 테이블의 필드를 확인하고 이에 해당하는 접근자
메소드를 동적으로 추가하는 User 모델 클래스의 기능으 ActiveRecord::Base클래스로 부터 상속되고 있다.
설정
트랙백
댓글
글
rails 플러그인 목록 주소(http://agilewebdevelopment.com/plugins/list)
http://agilewebdevelopment.com/plugins/list
설치법
ruby script/plugin install
http://daesan.com/svn/simple_rss
플러그인은 각 애플리케이션의 vendor/plugins/ 디렉토리에 저장된다.
설정
트랙백
댓글
글
각 mode별로 start 방법
ruby script/server -e development
ruby script/server -e test
ruby script/server -e production
-e가 생략되면 디폴트로 development 로 실행된다.
2. migration
rake dbLmigrate RAILS_ENV=development
rake dbLmigrate RAILS_ENV=test
rake dbLmigrate RAILS_ENV=production
설정
트랙백
댓글
글
migration 문법
create_table "groups" do |t|
t.column "name", :string, Lnull => false
end
2. default값 지정
create_table "users" do |t|
t.column "type", :string, Ldefault => "일반"
end
3. 문자열 컬럼 길이 지정
create_table "groups" do |t|
t.column "name", :string, Llimit => 10
end
4. 숫자컬럼 길이지정
create_table "people" do |t|
t.column "name", :string
t.column "height", :decimal, Lprecision => 5, Lscale => 2
end
5. boolean
boolean은 TINYINT로 지정이 되어 true(1), false(2) 로 저장이 된다.
6. 테이블명 변경
rename_table "groups", categories"
7. 인덱스 추가
add_index "groups", "name", :unique => true
remove_index "groups", "name"
8. 기타 SQL
마이그레이션에서 지원하지 않는 비표준 기능은 execute를 사용해라
execute "ALTER TABLE people ADD CONSTRAINT fk_person_group"